- สร้าง Flutter project ตั้งชื่อตามภาพ
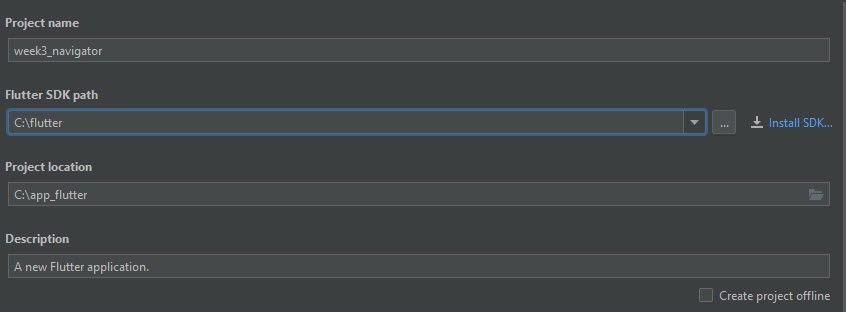
- แก้ไขไฟล์ Main.dart
import 'package:flutter/material.dart';
import 'package:week3_navigator/Page1.dart';
import 'package:week3_navigator/Page2.dart';
import 'package:week3_navigator/PageAbout.dart';
//หรือเขียนแบบลดรูปใช้ => ช่วยเช่น void main()=>runApp(MyApp());
void main(){
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Test',
theme: ThemeData(
primarySwatch: Colors.teal,
),
initialRoute: '/',
routes: {
'/':(context)=>Page1(title:'Page 1'),
'/page2':(context)=>Page2(title:'Page 2'),
'/ab':(context)=>PageAbout(title: 'Page About'),
},
);
}
}
- ให้สร้างไฟล์ โดยกดคลิกขวาที่ lib แล้ว new dart file ตั้งชื่อ Page1.dart แล้วพิมพ์โค้ดตามนี้
import 'package:flutter/material.dart';
class Page1 extends StatefulWidget {
Page1({Key key,this.title}):super(key:key);
final String title;
@override
_Page1State createState() => _Page1State();
}
class _Page1State extends State<Page1> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title:Text(widget.title),
),
body: Center(
child:Column(
children: <Widget>[
Image.network('https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcReXUMrZzOsVUptKhydvWXLi-SnrT7e_PZi_w&usqp=CAU'),
RaisedButton(
onPressed: (){
Navigator.pushNamed(context, '/page2');
},
child:Text('Go Page 2'),
),
],
),
),
);
}
}
- ให้สร้างไฟล์ โดยกดคลิกขวาที่ lib แล้ว new dart file ตั้งชื่อ Page2.dart แล้วพิมพ์โค้ดตามนี้
import 'package:flutter/material.dart';
class Page2 extends StatefulWidget {
Page2({Key key,this.title}):super(key:key);
final String title;
@override
_Page2State createState() => _Page2State();
}
class _Page2State extends State<Page2> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child:Column(
children: <Widget>[
Text('ยินดีต้อนรับสู่เพจ 2',style: TextStyle(color: Colors.red,fontSize: 26),),
RaisedButton(
onPressed: (){
Navigator.pushNamed(context, '/ab');
},
child:Text('ไป Page About'),
),
],
),
),
);
}
}
- ให้สร้างไฟล์ โดยกดคลิกขวาที่ lib แล้ว new dart file ตั้งชื่อ PageAbout.dart แล้วพิมพ์โค้ดตามนี้
import 'package:flutter/material.dart';
class PageAbout extends StatefulWidget {
PageAbout({Key key,this.title}):super(key:key);
final String title;
@override
_PageAboutState createState() => _PageAboutState();
}
class _PageAboutState extends State<PageAbout> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Text('ยินดีต้อนรับสู่เพจ About',style: TextStyle(color: Colors.red,fontSize: 26),),
),
);
}
}
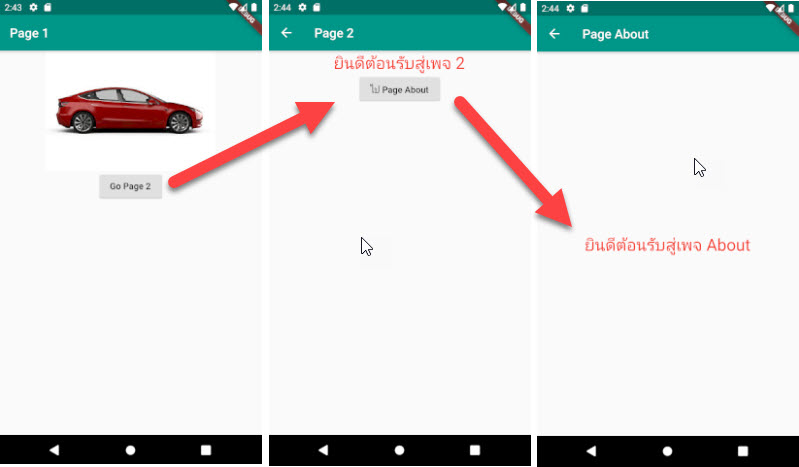
- ในหน้า PageAbout.dart เพิ่ม BottomNavigationBar
import 'package:flutter/material.dart';
class PageAbout extends StatefulWidget {
PageAbout({Key key,this.title}):super(key:key);
final String title;
@override
_PageAboutState createState() => _PageAboutState();
}
class _PageAboutState extends State<PageAbout> {
int _selectedIndex = 0;
static const TextStyle optionStyle = TextStyle(fontSize: 30, fontWeight: FontWeight.bold);
static const List<Widget> _widgetOptions = <Widget>[
Text('Index 0: Home',style: optionStyle,),
Text('Index 1: Business',style: optionStyle,),
Image(image: NetworkImage('https://flutter.github.io/assets-for-api-docs/assets/widgets/owl.jpg'),
),];
void _onItemTapped(int index) {
setState(() {_selectedIndex = index; });
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: _widgetOptions.elementAt(_selectedIndex),
),
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(icon: Icon(Icons.home),label: 'home'),
BottomNavigationBarItem(icon: Icon(Icons.business),label: 'business'),
BottomNavigationBarItem(icon: Icon(Icons.thumb_up),label: 'thumb_up'),
],
currentIndex: _selectedIndex,
selectedItemColor: Colors.red[800],
onTap: _onItemTapped,
),
);
}
}

- สร้างแอพใหม่ขึ้นมาใส่ชื่อ week3_randomnumber
- แก้ไขโค้ดหน้า main.dart
import 'dart:math';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
String random_number(){
var rgn=new Random();
String num=rgn.nextInt(9).toString();
return num;
}
class _MyHomePageState extends State<MyHomePage> {
final txt1=TextEditingController();
int _counter = 0;
String _answer=random_number();
String _result="";
void _guess_number(){
setState(() {
if(_answer==txt1.text){
_result="ถูกต้อง";
}
else{
//_result="คำตอบผิด ที่ถูกคือ :"+_answer;
_result="คำตอบผิด ที่ถูกคือ : $_answer";
}
});
}
void _new_game(){
setState(() {
_result = 'เดาตัวเลข';
_answer = random_number();
txt1.text = '';
});
}
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
TextField(
controller: txt1,
keyboardType: TextInputType.number,
),
Text(
// "ans : $_result"
_result
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
RaisedButton(
color: Colors.red,
onPressed: (){
//print(random_number());
// print(txt1.text);
_guess_number();
},
child:Text('ส่งคำตอบ'),
),
Padding(padding: EdgeInsets.all(5)),
RaisedButton(
color: Colors.blue,
onPressed: (){
_new_game();
},
child:Text('เริ่มใหม่'),
),
],
),
],
),
),
);
}
}
